As software developers, it’s critical we build security into our code from the very start of the development process. Poor coding practices can introduce vulnerabilities that put users at risk. In this post, I’ll outline some of the most common security issues developers face and effective ways to avoid them.
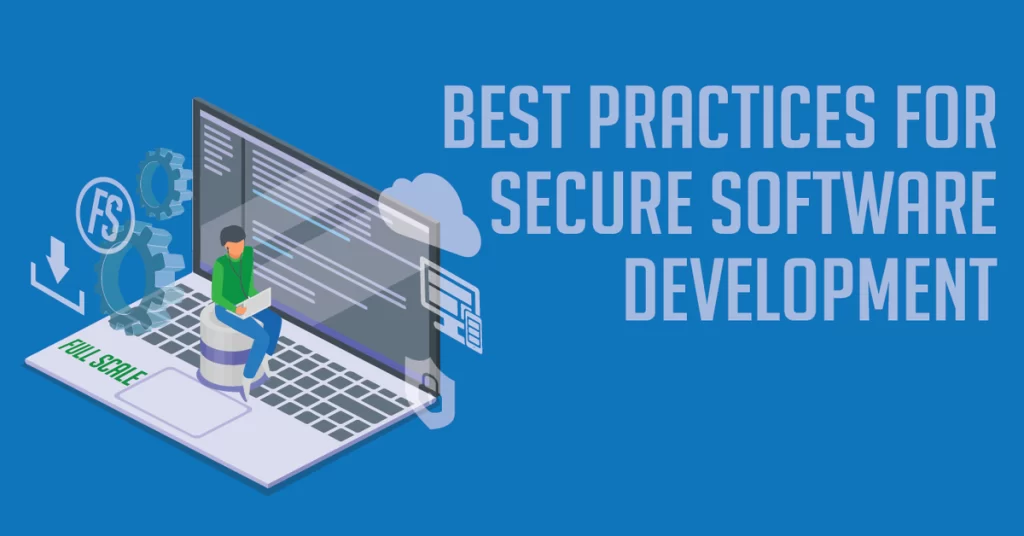
Content
Input Validation
One of the primary ways attackers compromise systems is through invalid or malicious user input. Failing to validate input is one of the most frequent causes of software vulnerabilities. Proper validation of all user-supplied data is a must before using it within an application.
This includes validating data types, lengths, syntax and anything else that could be exploited. Never trust any data received from the client-side.
Some best practices for input validation include:
- Check for correct data types (strings, integers, floats, etc.) and lengths for all input fields
- Filter out restricted characters like HTML tags, script code or odd encodings
- Validate against schemas or whitelist expected values rather than blacklisting “bad” values
- Perform validation on the server-side as client-side validation can be bypassed
Cross-Site Scripting (XSS)
XSS occurs when an application includes untrusted data in its output without validating or encoding it. This allows attackers to execute scripts in a victim’s browser to hijack user sessions, hijack the user’s browser using pop-ups or redirects, or modifying presentation of content. XSS flaws continue to be one of the most prevalent web application vulnerabilities.
To prevent XSS, make sure all dynamic output is properly encoded before inclusion. Default encoding schemes include HTML encoding for HTML content, URL encoding for URLs and so on. Also consider Content Security Policy headers to block dangerous scripts from executing.
Code Injection
Similar to XSS, code injection flaws allow attackers to inject and execute arbitrary code on the server. This includes SQL injection, OS command injection, XML injection and path traversal. Attackers exploit these flaws to alter back-end database contents, bypass authentication and authorization, or gain remote code execution on the system.
The root cause is again improper sanitization of untrusted data used in a code execution context like SQL queries, system calls or XML parsers. Always use parameterized queries, sanitize input data and avoid direct injections whenever possible during software development.
Authentication and Authorization
Poor or missing authentication and authorization is one of the easiest paths for attackers to gain access. Applications should have solid credential management and session handling in place. Some security best practices include:
- Use industry-standard techniques like salted hashed passwords
- Set secure authentication cookies with the HttpOnly flag
- Enforce account lockouts after failed login attempts
- Implement role-based access control for functional areas
- Log and audit all authentication/authorization activities
- Validate user permissions on every request
Following foundational security practices during software development like those outlined above can help eliminate entire classes of vulnerabilities. Of course, applications also need ongoing security testing and maintenance. But building with security in mind from the start significantly reduces future risks for both developers and users. Understanding the most common weaknesses is the first step towards secure coding.
Protecting APIs
Modern applications frequently expose APIs that power features like native mobile apps, integration with third-party services and more. Yet many developers overlook securing these interfaces. Ensure any APIs are:
- Authenticated like a user to establish caller identity
- Authorization checks requests against predefined roles
- Use SSL/TLS to encrypt traffic
- Validate schema of input data
- Limit rate of requests
- Consider versioning APIs for backward compatibility
APIs that lacked basic authentication and input validation have been exploited in many high-profile breaches. Taking security into account throughout the software development process helps deliver more robust applications and better protects user data.
Conclusion
In conclusion, following secure coding practices provides multiple layers of protection while avoiding entire categories of vulnerabilities. With a bit of awareness and diligence during development, many issues can be avoided altogether.
Developers play a critical role in security, so prioritizing these basics yields major wins for both themselves and their users long-term. Staying up to date on the latest risks also helps shore up any weaknesses before they’re discovered. Overall, secure software relies on secure code – so security should not be an afterthought.
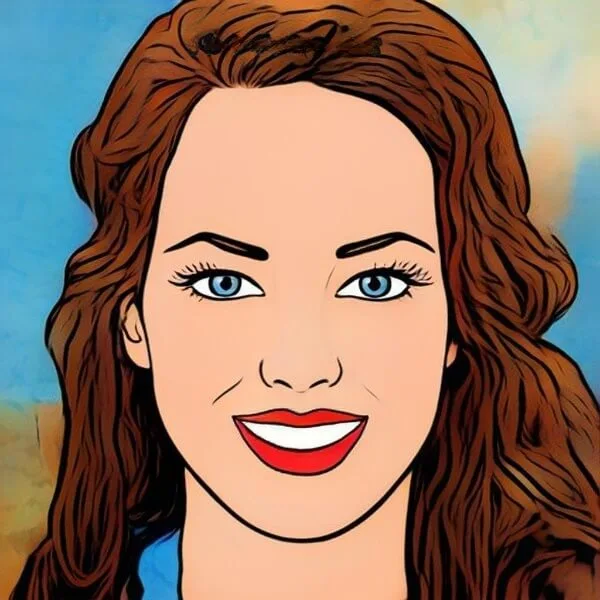
I’m Kelly Hood! I blog about tech, how to use it, and what you should know. I love spending time with my family and sharing stories of the day with them.